* 도서 관리 페이지 예제
01 데이터베이스
1) BOOKSTORE 테이블
2) BNO(PK), BNAME, AUTHOR, PUBLISHER, PRICE, CNT 컬럼
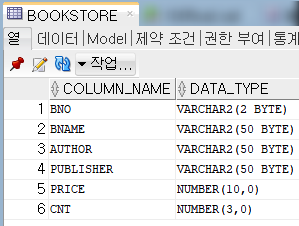
3) BOOKLIST 테이블
4) LNO(PK), BNAME, PRICE, CNT, TOT 컬럼
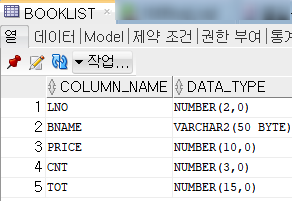
5) LOGIN 테이블
6) ID, PW 컬럼

02 TOP / Top.jsp
1) 도서 목록, 도서 검색, 구입 목록, 도서 추가, 도서 수정으로 가는 링크 (어느 페이지에서도 뜨도록)
2) header 이미지의 아이콘을 누르면 메인 페이지로 이동
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>H BookStore</title>
<link href="css/Style.css" rel="stylesheet" type="text/css">
</head>
<body>
<header>
<h1><a href="Main.jsp"><img src="image/bookicn.png" width="40px" height="40px"></a> H - B o o k S t o r e</h1>
</header>
<nav>
<a href="Output.jsp">도서 목록</a>  
<a href="SearchForm.jsp">도서 검색</a>  
<a href="BuyList.jsp">구입 목록</a>  
<a href="Login_i.do">도서 추가</a>  
<a href="Login_m.do">도서 수정</a>  
</nav>
</body>
</html>
03 Footer / Footer.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>H BookStore</title>
<link href="css/Style.css" rel="stylesheet" type="text/css">
</head>
<body>
<footer>
대한민국 경기도 내 어딘가<br>
Developer by Hong.s
</footer>
</body>
</html>
04 Main / Main.jsp
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ include file="Top.jsp" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>H BookStore</title>
<link href="css/Style.css" rel="stylesheet" type="text/css" />
</head>
<body>
<section>
<img src="image/book.jpg" width="100%" height="400px">
</section>
</body>
<%@ include file="Footer.jsp" %>
</html>

05 도서 목록 / Output.jsp
1) BOOKSTORE 테이블에 있는 데이터들 모두 출력
2) 구입 링크를 누르면 Buy.jsp로 이동 (bno, bname, price, cnt 값을 가지고 이동)
<%@page import="java.sql.DriverManager"%>
<%@page import="java.sql.ResultSet"%>
<%@page import="java.sql.PreparedStatement"%>
<%@page import="java.sql.Connection"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ include file="Top.jsp" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>H BookStore</title>
<link href="css/Style.css" rel="stylesheet" type="text/css">
</head>
<body>
<div class="container">
<h3><span>도서 목록</span> 페이지</h3>
<hr width="100%">
<p>
<%!
String bno, bname, author, publisher;
int price, cnt;
%>
<%
request.setCharacterEncoding("utf-8");
Connection con;
PreparedStatement psmt;
ResultSet res;
String driver="oracle.jdbc.driver.OracleDriver";
String url="jdbc:oracle:thin:@localhost:1521:xe";
Class.forName(driver);
con=DriverManager.getConnection(url, "아이디", "비밀번호");
String sql="select * from bookstore order by bno";
psmt=con.prepareStatement(sql);
res=psmt.executeQuery();
%>
<table border="1">
<tr><th>번호</th><th>도서명</th><th>저자</th><th>출판사</th><th>가격</th><th>수량</th><th>구입</th></tr>
<%
while(res.next()){
bno = res.getString("bno");
bname = res.getString("bname");
author = res.getString("author");
publisher = res.getString("publisher");
price = res.getInt("price");
cnt = res.getInt("cnt");
%>
<tr><td><%=bno %></td><td><%=bname %></td><td><%=author %></td><td><%=publisher %></td><td><%=price %></td><td><%=cnt %></td>
<td><a href="Buy.jsp?bno=<%=bno %>&bname=<%=bname %>&price=<%=price %>&cnt=<%=cnt %>">구입</a></td>
</tr>
<%
}
%>
</table>
</div>
</body>
<%@ include file="Footer.jsp" %>
</html>

06 - ① 도서 구입 폼 / Buy.jsp
1) bno, bname, price, cnt 값을 받아 온 상태
2) Buy.do로 보내기 위해 HttpSession을 사용하여 위의 값 저장(setAttribute)
3) 구입하고자 하는 수량을 입력 받기(b_cnt)
4) bno: 책 번호, bname: 도서명, price: 가격, cnt: 해당 도서의 재고 수, b_cnt: 구입하고자 하는 수량
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ include file="Top.jsp" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>H BookStore</title>
<link href="css/Style.css" rel="stylesheet" type="text/css">
</head>
<body>
<div class="container">
<h3><span>도서 구입</span> 페이지</h3>
<hr width="100%">
<p>
<%
request.setCharacterEncoding("utf-8");
String bno = request.getParameter("bno");
String bname = request.getParameter("bname");
int price = Integer.parseInt(request.getParameter("price"));
int cnt = Integer.parseInt(request.getParameter("cnt"));
HttpSession hs = request.getSession();
hs.setAttribute("bno", bno);
hs.setAttribute("bname", bname);
hs.setAttribute("price", price);
hs.setAttribute("cnt", cnt);
%>
<form action="Buy.do" method="post">
<table>
<tr><td>도서명</td><td><%=bname %></td></tr>
<tr><td>가격</td><td><%=price %></td></tr>
<tr><td>수량</td><td><input type="number" name="b_cnt"></td></tr>
</table>
<p><p>
<input type="submit" value="구입">
<input type="reset" value="취소">
</form>
</div>
</body>
<%@ include file="Footer.jsp" %>
</html>

06 - ② 도서 구입 서블릿 / FrontController.java(Buy.do)
1) HttpSession에 저장했던 bno, bname, price, cnt 값 가져오기(getAttribute)
2) BOOKLIST에 추가할 합계와 구입한 수량 저장
3) tcnt = 구입 전 수량 - 구입할 수량 (남은 재고 수)
4) BOOKSTORE에서 bno에 해당하는 cnt 수정(구입한 만큼 (-)연산)
5) BOOKLIST에 날짜, 책이름, 가격, 구입한 수량, 합계 추가
6) 리스트 번호는 전역변수로 int lno=0; 설정 후 Buy.do 소스코드에 들어올 때마다 lno 1씩 증가 후 추가
7) BOOKLIST에 성공적으로 데이터들을 추가하면 BuyList.jsp로 이동
/*
* 중략
*/
else if(fname.equals("/Buy.do")) {
LocalDateTime now = LocalDateTime.now();
String formatedNow = now.format(DateTimeFormatter.ofPattern("yy-MM-dd HH:mm:ss"));
HttpSession hs = request.getSession();
String bno = (String)hs.getAttribute("bno");
String bdate = formatedNow;
String bname = (String)hs.getAttribute("bname");
int price = (Integer)hs.getAttribute("price");
int b_cnt = Integer.parseInt(request.getParameter("b_cnt"));
int tot = price * b_cnt;
int cnt = (Integer)hs.getAttribute("cnt");
int tcnt = cnt - b_cnt;
String sql="update bookstore set cnt=? where bno=?";
try {
psmt=con.prepareStatement(sql);
psmt.setInt(1, tcnt);
psmt.setString(2, bno);
psmt.executeUpdate();
} catch (SQLException e) {
e.printStackTrace();
}
String sql2="insert into booklist values(?,?,?,?,?)";
try {
psmt=con.prepareStatement(sql2);
psmt.setString(1, bdate);
psmt.setString(2, bname);
psmt.setInt(3, price);
psmt.setInt(4, b_cnt);
psmt.setInt(5, tot);
int k=psmt.executeUpdate();
if(k==1)
response.sendRedirect("BuyList.jsp");
} catch (SQLException e) {
e.printStackTrace();
}
}
/*
* 중략
*/
06 - ③ 도서 구입 목록 / BuyList.jsp
1) BOOKLIST 테이블에 있는 데이터들을 날짜 내림차순으로 모두 출력
<%@page import="java.sql.DriverManager"%>
<%@page import="java.sql.ResultSet"%>
<%@page import="java.sql.PreparedStatement"%>
<%@page import="java.sql.Connection"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ include file="Top.jsp" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>H BookStore</title>
<link href="css/Style.css" rel="stylesheet" type="text/css">
</head>
<body>
<div class="container">
<h3><span>구입 목록</span> 페이지</h3>
<hr width="100%">
<p>
<%!
int price, cnt, tot;
String bdate, bname;
%>
<%
request.setCharacterEncoding("utf-8");
Connection con;
PreparedStatement psmt;
ResultSet res;
String driver="oracle.jdbc.driver.OracleDriver";
String url="jdbc:oracle:thin:@localhost:1521:xe";
Class.forName(driver);
con=DriverManager.getConnection(url, "아이디", "비밀번호");
String sql="select * from booklist order by bdate desc";
psmt=con.prepareStatement(sql);
res=psmt.executeQuery();
%>
<table border="1">
<tr><th>날짜</th><th>도서명</th><th>가격</th><th>수량</th><th>합계</th></tr>
<%
while(res.next()){
bdate = res.getString("bdate");
bname = res.getString("bname");
price = res.getInt("price");
cnt = res.getInt("cnt");
tot = res.getInt("tot");
%>
<tr><td><%=bdate %></td><td><%=bname %></td><td><%=price %></td><td><%=cnt %></td><td><%=tot %></td></tr>
<%
}
%>
</table>
</div>
</body>
<%@ include file="Footer.jsp" %>
</html>
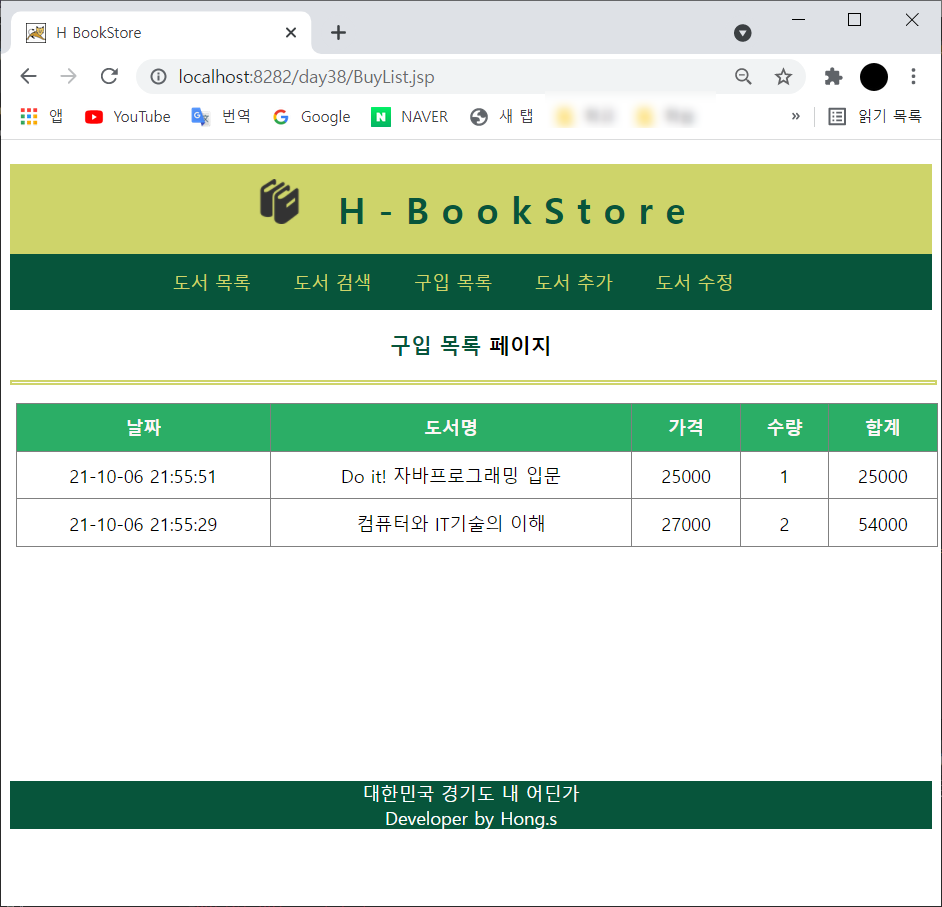
07 - ① 도서 검색 폼/ SearchForm.jsp
1) 검색할 도서명을 입력 받아 Search.jsp로 전송
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ include file="Top.jsp" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>H BookStore</title>
<link href="css/Style.css" rel="stylesheet" type="text/css">
</head>
<body>
<div class="container">
<h3><span>도서 검색</span> 페이지</h3>
<hr width="100%">
<p>
<form action="Search.jsp" method="post">
검색할 도서의 이름을 입력하세요<br><br>
도서명: <input type="text" name="s_bname">
<p>
<input type="submit" value="검색">
<input type="reset" value="취소">
</form>
</div>
</body>
<%@ include file="Footer.jsp" %>
</html>
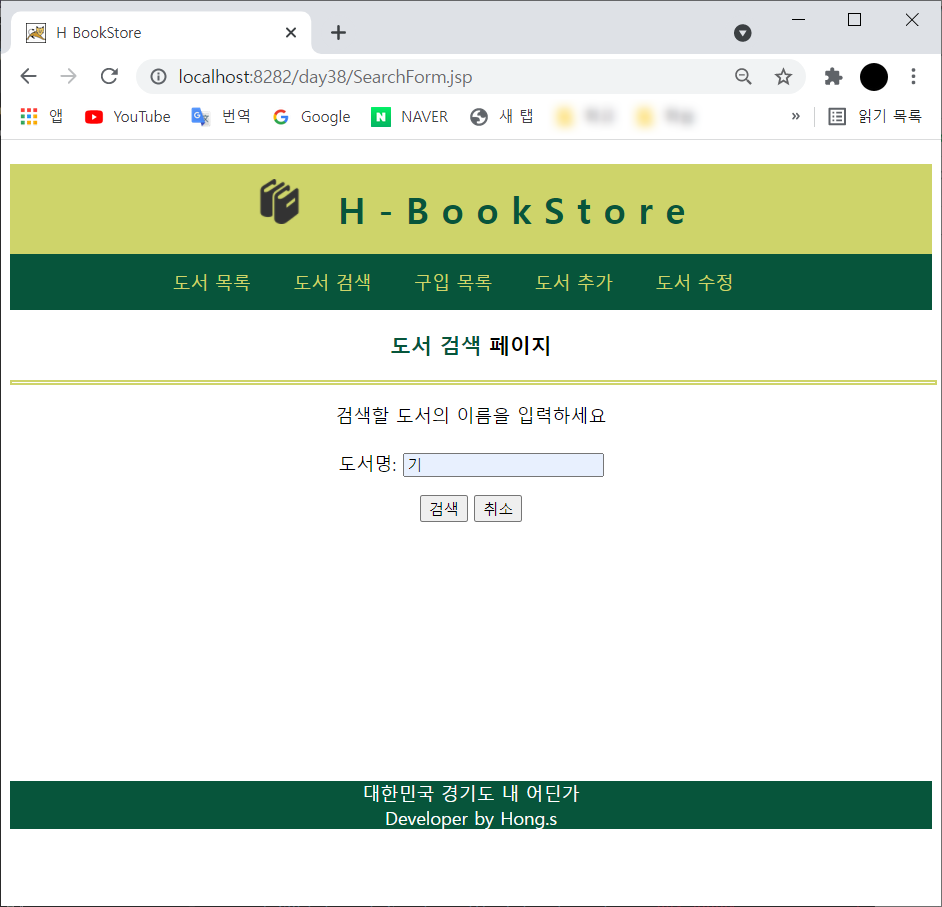
07 - ② 도서 검색 / Search.jsp
1) 검색할 도서명이 포함된 모든 도서 출력 SELECT * FROM BOOKSTORE WHERE BNAME LIKE '%검색도서명%';
<%@page import="java.sql.DriverManager"%>
<%@page import="java.sql.ResultSet"%>
<%@page import="java.sql.PreparedStatement"%>
<%@page import="java.sql.Connection"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ include file="Top.jsp" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>H BookStore</title>
<link href="css/Style.css" rel="stylesheet" type="text/css">
</head>
<body>
<div class="container">
<h3><span>도서 검색</span> 페이지</h3>
<hr width="100%">
<p>
<%!
String s_bname, bno, author, publisher, bname;
int price, cnt;
%>
<%
request.setCharacterEncoding("utf-8");
Connection con;
PreparedStatement psmt;
ResultSet res;
String driver="oracle.jdbc.driver.OracleDriver";
String url="jdbc:oracle:thin:@localhost:1521:xe";
Class.forName(driver);
con=DriverManager.getConnection(url, "아이디", "비밀번호");
s_bname = request.getParameter("s_bname");
String sql = "select * from bookstore where bname like ?";
psmt=con.prepareStatement(sql);
psmt.setString(1, "%" + s_bname + "%");
res=psmt.executeQuery();
%>
<table border="1">
<tr><th>번호</th><th>도서명</th><th>저자</th><th>출판사</th><th>가격</th><th>수량</th></tr>
<%
while(res.next()){
bno = res.getString("bno");
bname = res.getString("bname");
author = res.getString("author");
publisher = res.getString("publisher");
price = res.getInt("price");
cnt = res.getInt("cnt");
%>
<tr><td><%=bno %></td><td><%=bname %></td><td><%=author %></td><td><%=publisher %></td><td><%=price %></td><td><%=cnt %></td></tr>
<%
}
%>
</table>
</div>
</body>
<%@ include file="Footer.jsp" %>
</html>
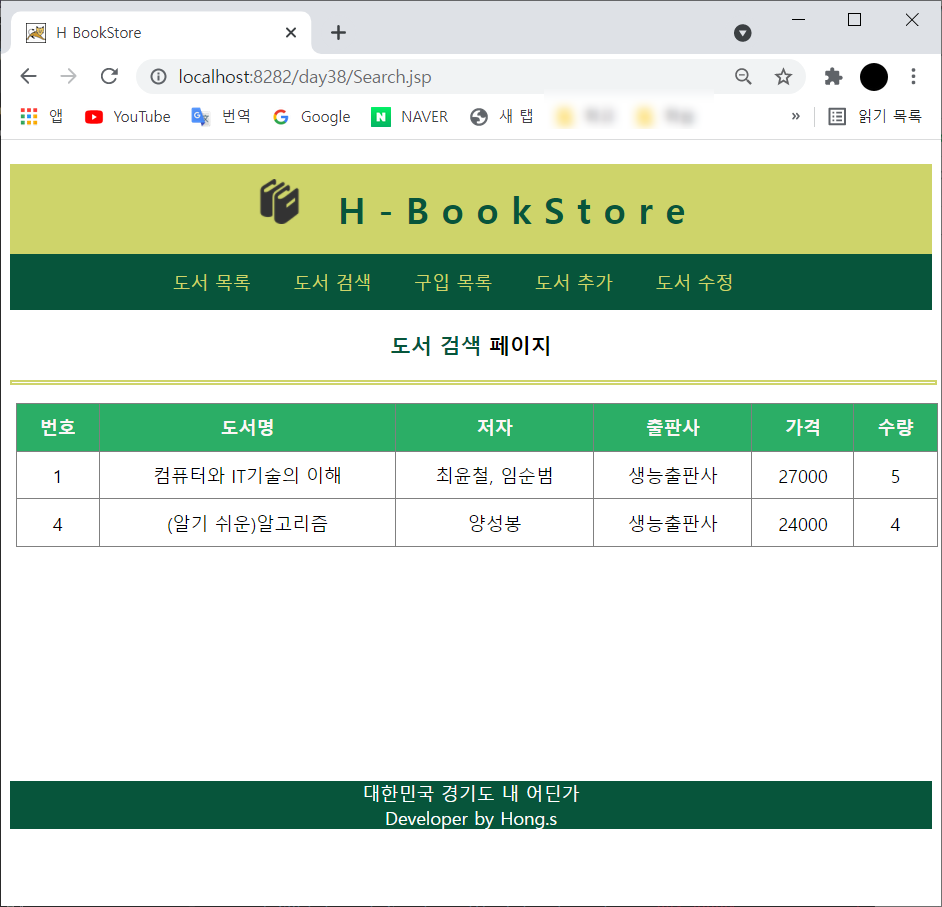
08 - ① 도서 추가 > 로그인 서블릿 / FrontController.java(Login_i.do)
1) 도서 추가와 도서 수정은 관리자 로그인이 필요
2) 도서 추가 버튼을 누른 후 로그인을 하면 index=1로 변경 (flag) 후 Login.jsp로 이동
/*
* 중략
*/
else if(fname.equals("/Login_i.do")) {
index=1;
response.sendRedirect("Login.jsp");
}
/*
* 중략
*/
08 - ② 관리자 로그인 폼 / Login.jsp
1) ID와 PW 입력 후 Success.do로 이동
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ include file="Top.jsp" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>H BookStore</title>
<link href="css/Style.css" rel="stylesheet" type="text/css">
</head>
<body>
<div class="container">
<h3><span>관리자 로그인</span> 페이지</h3>
<hr width="100%">
<p>
<form action="Success.do" method="post">
ID <input type="text" name="id"><p>
PW <input type="password" name="pw"><p>
<p><p>
<input type="submit" value="로그인">
<input type="reset" value="취소">
</form>
</div>
</body>
<%@ include file="Footer.jsp" %>
</html>
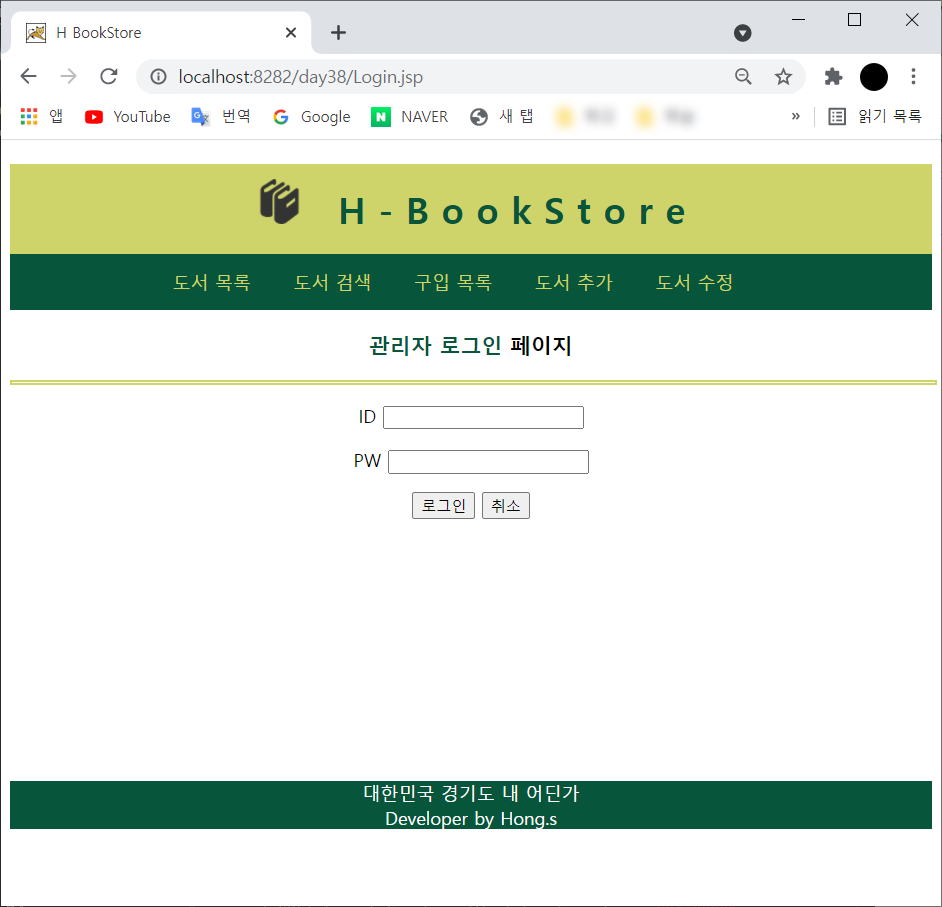
08 - ③ 로그인 확인 / FrontController.java(Success.do)
1) LOGIN 테이블에서 ID와 PW 모두 일치하는지 확인
2) 일치하면 index 비교, index가 1이면 도서 추가(Insert.jsp)로 이동
/*
* 중략
*/
else if(fname.equals("/Success.do")) {
String id = request.getParameter("id");
String pw = request.getParameter("pw");
String sql="select * from login where id=? and pw=?";
try {
psmt=con.prepareStatement(sql);
psmt.setString(1, id);
psmt.setString(2, pw);
res=psmt.executeQuery();
if(res.next()) {
if(index==1)
response.sendRedirect("Insert.jsp");
else if(index==2)
response.sendRedirect("Admin_Output.jsp");
}
else{
response.sendRedirect("Login.jsp");
}
} catch (SQLException e) {
e.printStackTrace();
}
}
/*
* 중략
*/
08 - ④ 도서 추가 폼 / Insert.jsp
1) BOOKSTORE에 들어갈 데이터 입력받아 Insert.do로 전송
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ include file="Top.jsp" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>H BookStore</title>
<link href="css/Style.css" rel="stylesheet" type="text/css">
</head>
<body>
<div class="container">
<h3><span>도서 추가</span> 페이지</h3>
<hr width="100%">
<p>
<form action="Insert.do" method="post">
<table>
<tr><td>도서번호</td><td><input type="text" name="bno"></td></tr>
<tr><td>도서명</td><td><input type="text" name="bname"></td></tr>
<tr><td>저자</td><td><input type="text" name="author"></td></tr>
<tr><td>출판사</td><td><input type="text" name="publisher"></td></tr>
<tr><td>가격</td><td><input type="text" name="price"></td></tr>
<tr><td>수량</td><td><input type="text" name="cnt"></td></tr>
</table>
<p><p>
<input type="submit" value="추가">
<input type="reset" value="취소">
</form>
</div>
</body>
<%@ include file="Footer.jsp" %>
</html>

08 - ⑤ 도서 추가 서블릿 / FrontController.java(Insert.do)
1) 넘겨받은 데이터를 BOOKSTORE에 추가
2) 성공적으로 추가하면 Admin_Output.jsp로 이동
/*
* 중략
*/
if(fname.equals("/Insert.do")) {
String bno = request.getParameter("bno");
String bname = request.getParameter("bname");
String author = request.getParameter("author");
String publisher = request.getParameter("publisher");
int price = Integer.parseInt(request.getParameter("price"));
int cnt = Integer.parseInt(request.getParameter("cnt"));
String sql="insert into bookstore values(?,?,?,?,?,?)";
try {
psmt=con.prepareStatement(sql);
psmt.setString(1, bno);
psmt.setString(2, bname);
psmt.setString(3, author);
psmt.setString(4, publisher);
psmt.setInt(5, price);
psmt.setInt(6, cnt);
int k = psmt.executeUpdate();
if(k==1)
response.sendRedirect("Admin_Output.jsp");
} catch (SQLException e) {
e.printStackTrace();
}
}
/*
* 중략
*/
09 관리자 도서 목록 / Admin_Output.jsp
1) BOOKSTORE에 있는 데이터들을 모두 출력
2) 수정과 삭제로 가기 위한 링크(bno, bname 값을 가지고 이동)
<%@page import="java.sql.DriverManager"%>
<%@page import="java.sql.ResultSet"%>
<%@page import="java.sql.PreparedStatement"%>
<%@page import="java.sql.Connection"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ include file="Top.jsp" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>H BookStore</title>
<link href="css/Style.css" rel="stylesheet" type="text/css">
</head>
<body>
<div class="container">
<h3><span>도서 목록</span> 페이지</h3>
<hr width="100%">
<p>
<%!
String bno, bname, author, publisher;
int price, cnt;
%>
<%
request.setCharacterEncoding("utf-8");
Connection con;
PreparedStatement psmt;
ResultSet res;
String driver="oracle.jdbc.driver.OracleDriver";
String url="jdbc:oracle:thin:@localhost:1521:xe";
Class.forName(driver);
con=DriverManager.getConnection(url, "아이디", "비밀번호");
String sql="select * from bookstore order by bno";
psmt=con.prepareStatement(sql);
res=psmt.executeQuery();
%>
<table border="1">
<tr><th>번호</th><th>도서명</th><th>저자</th><th>출판사</th><th>가격</th><th>수량</th><th>수정</th><th>삭제</th></tr>
<%
while(res.next()){
bno = res.getString("bno");
bname = res.getString("bname");
author = res.getString("author");
publisher = res.getString("publisher");
price = res.getInt("price");
cnt = res.getInt("cnt");
%>
<tr><td><%=bno %></td><td><%=bname %></td><td><%=author %></td><td><%=publisher %></td><td><%=price %></td><td><%=cnt %></td>
<td><a href="Modify.jsp?m_bno=<%=bno %>&m_bname=<%=bname %>">수정</a></td>
<td><a href="Delete.do?d_bno=<%=bno %>">삭제</a></td>
</tr>
<%
}
%>
</table>
</div>
</body>
<%@ include file="Footer.jsp" %>
</html>
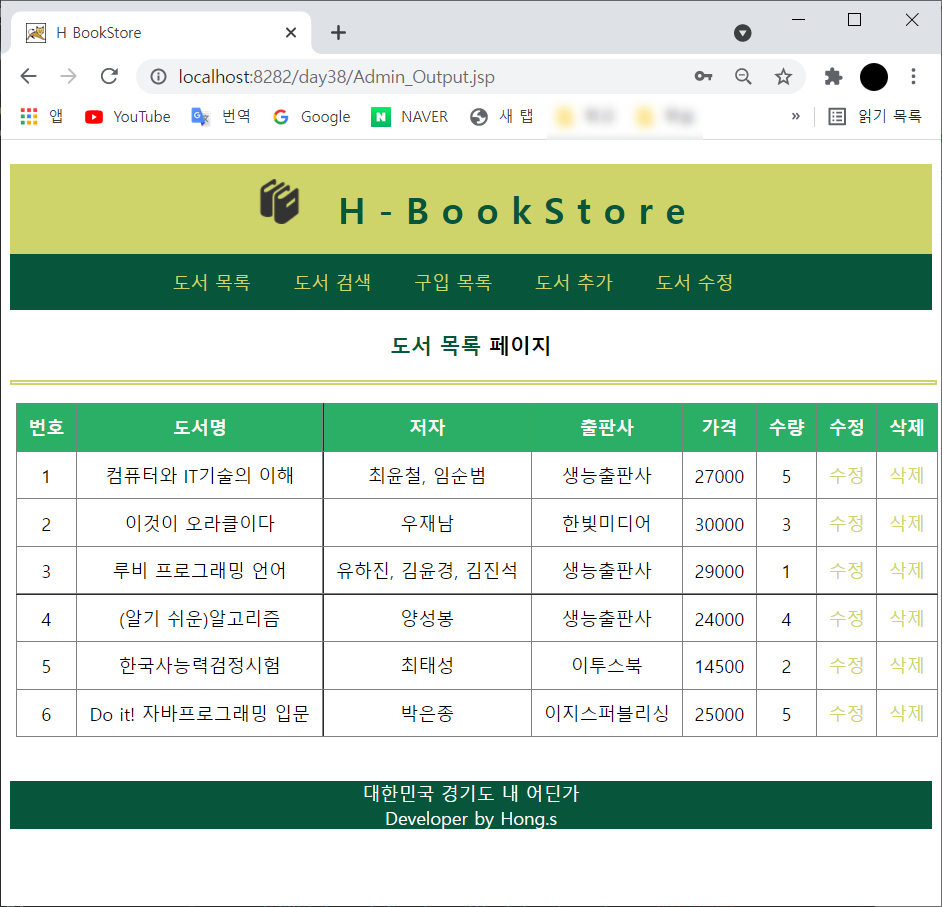
10 - ① 도서 수정 > 로그인 서블릿 / FrontController.java(Login_m.do)
1) 도서 추가와 도서 수정은 관리자 로그인이 필요
2) 도서 수정 버튼을 누른 후 로그인을 하면 index=2로 변경 (flag) 후 Login.jsp로 이동
/*
* 중략
*/
else if(fname.equals("/Login_m.do")) {
index=2;
response.sendRedirect("Login.jsp");
}
/*
* 중략
*/
10 - ② 관리자 로그인 폼 / Login.jsp (08-②)
10 - ③ 로그인 확인 / FrontController.java(Success.do) (08-③)
1) LOGIN 테이블에서 ID와 PW 모두 일치하는지 확인
2) 일치하면 index 비교, index가 2이면 관리자 도서 목록 페이지(Admin_Output.jsp)로 이동
10 - ④ 도서 수정 폼 / Modify.jsp
1) Admin_Output.jsp에서 수정 링크를 누르면 Modify.jsp로 이동
2) bno와 bname 값은 유지하고 저자, 출판사, 가격, 수량 수정 가능
3) bno와 bname 값은 HttpSession을 이용해 저장(setAttribute)
4) 수정 버튼을 누르면 Modify.do로 전송
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ include file="Top.jsp" %>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>H BookStore</title>
<link href="css/Style.css" rel="stylesheet" type="text/css">
</head>
<body>
<%
String m_bno = request.getParameter("m_bno");
String m_bname = request.getParameter("m_bname");
%>
<div class="container">
<h3><span>도서 수정</span> 페이지</h3>
<hr>
<p>
<form action="Modify.do" method="post">
<table>
<tr><td>도서번호</td><td><%=m_bno %></td></tr>
<tr><td>도서명</td><td><%=m_bname %></td></tr>
<tr><td>저자</td><td><input type="text" name="m_author"></td></tr>
<tr><td>출판사</td><td><input type="text" name="m_publisher"></td></tr>
<tr><td>가격</td><td><input type="text" name="m_price"></td></tr>
<tr><td>수량</td><td><input type="text" name="m_cnt"></td></tr>
</table>
<p><p>
<%
HttpSession hs = request.getSession();
hs.setAttribute("m_bno", m_bno);
hs.setAttribute("m_bname", m_bname);
%>
<input type="submit" value="수정">
<input type="reset" value="취소">
</form>
</div>
</body>
<%@ include file="Footer.jsp" %>
</html>
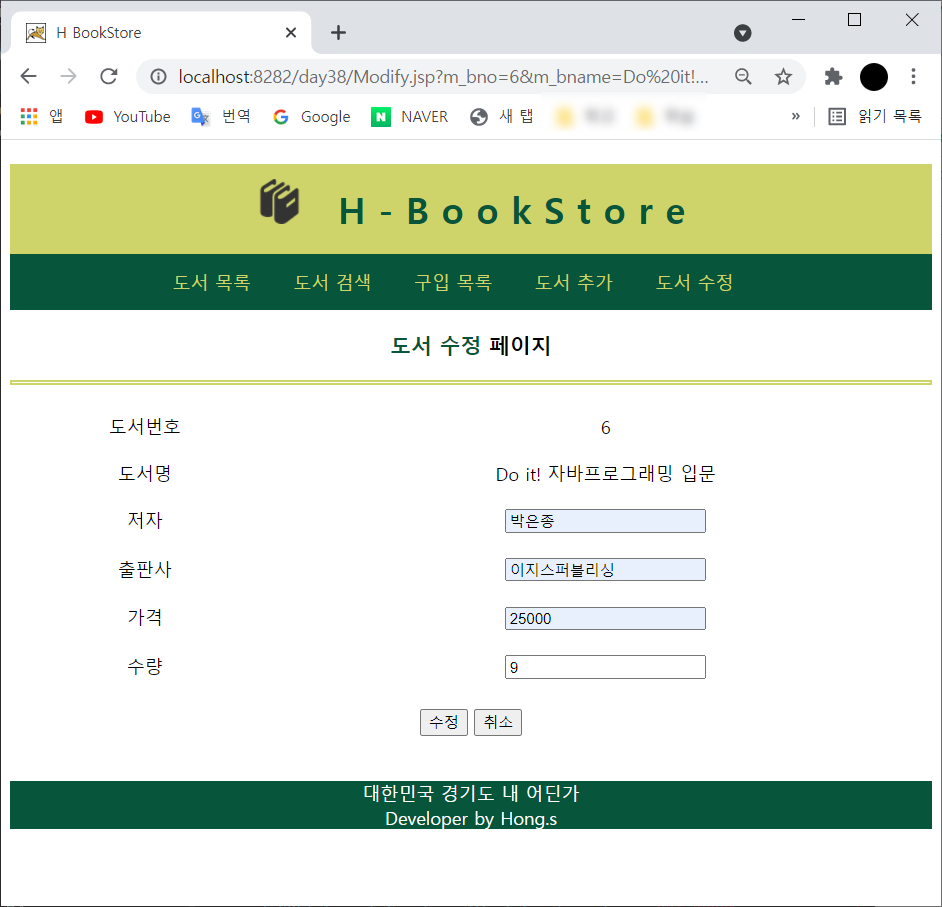
10 - ⑤ 도서 수정 서블릿 / FrontController.java(Modify.do)
1) HttpSession을 이용해 bno와 bname 값 가져오기(getAttribute)
2) BOOKSTORE 테이블에서 저자, 출판사, 가격, 수량 변경
3) 변경에 성공하면 Admin_Output.jsp로 이동
/*
* 중략
*/
else if(fname.equals("/Modify.do")) {
HttpSession hs = request.getSession();
String bno = (String)hs.getAttribute("m_bno");
String bname = (String)hs.getAttribute("m_bname");
String author = request.getParameter("m_author");
String publisher = request.getParameter("m_publisher");
int price = Integer.parseInt(request.getParameter("m_price"));
int cnt = Integer.parseInt(request.getParameter("m_cnt"));
String sql="update bookstore set author=?, publisher=?, price=?, cnt=? where bno=? and bname=?";
try {
psmt=con.prepareStatement(sql);
psmt.setString(1, author);
psmt.setString(2, publisher);
psmt.setInt(3, price);
psmt.setInt(4, cnt);
psmt.setString(5, bno);
psmt.setString(6, bname);
int k=psmt.executeUpdate();
if(k==1)
response.sendRedirect("Admin_Output.jsp");
} catch (SQLException e) {
e.printStackTrace();
}
}
/*
* 중략
*/
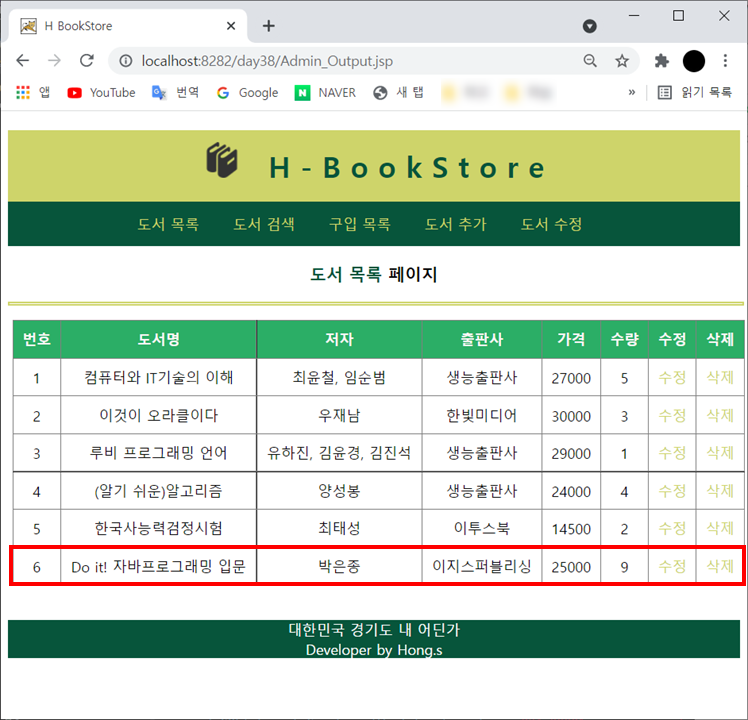
11 도서 삭제 / FrontController.java(Delete.do)
1) Admin_Output.jsp에서 삭제 링크를 누르면 Delete.do로 이동
2) 해당하는 bno의 데이터를 삭제
3) 성공적으로 삭제하면 Admin_Output.jsp로 이동
/*
* 중략
*/
else if(fname.equals("/Delete.do")) {
String d_bno = request.getParameter("d_bno");
String sql="delete from bookstore where bno=?";
try {
psmt=con.prepareStatement(sql);
psmt.setString(1, d_bno);
int k=psmt.executeUpdate();
if(k==1)
response.sendRedirect("Admin_Output.jsp");
} catch (SQLException e) {
e.printStackTrace();
}
}
/*
* 중략
*/
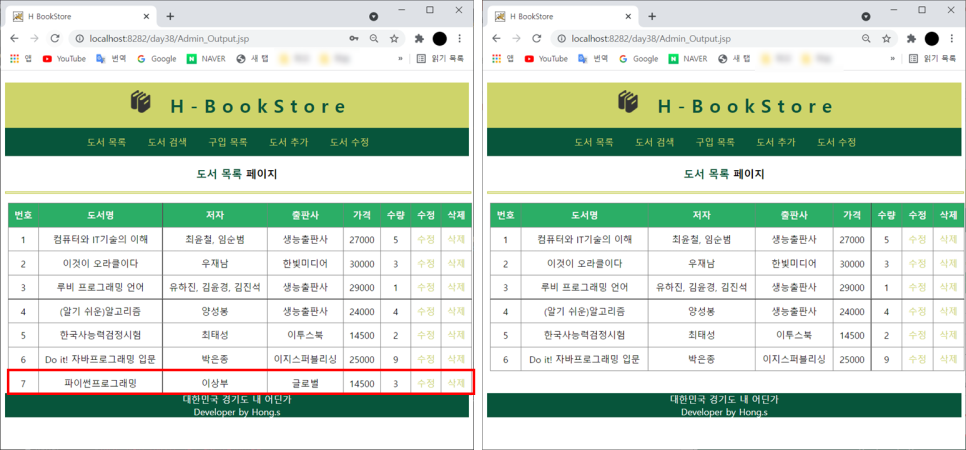
12 FrontController.java (Servlet) - 확장자 패턴 이용
package com.book;
import java.io.IOException;
import java.io.PrintWriter;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
/**
* Servlet implementation class FrontController
*/
@WebServlet("*.do")
public class FrontController extends HttpServlet {
private static final long serialVersionUID = 1L;
int index=0;
/**
* @see HttpServlet#HttpServlet()
*/
public FrontController() {
super();
// TODO Auto-generated constructor stub
}
/**
* @see HttpServlet#doGet(HttpServletRequest request, HttpServletResponse response)
*/
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("utf-8");
response.setContentType("text/html;charset=utf-8");
String uri=request.getRequestURI();
String conpath=request.getContextPath();
String fname=uri.substring(conpath.length());
Connection con=null;
PreparedStatement psmt;
ResultSet res;
String driver="oracle.jdbc.driver.OracleDriver";
String url="jdbc:oracle:thin:@localhost:1521:xe";
try {
Class.forName(driver);
con = DriverManager.getConnection(url, "아이디", "비밀번호");
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
}
if(fname.equals("/Insert.do")) {
**
}
else if(fname.equals("/Delete.do")) {
**
}
else if(fname.equals("/Modify.do")) {
**
}
else if(fname.equals("/Login_i.do")) {
**
}
else if(fname.equals("/Login_m.do")) {
**
}
else if(fname.equals("/Success.do")) {
**
}
else if(fname.equals("/Buy.do")) {
**
}
}
/**
* @see HttpServlet#doPost(HttpServletRequest request, HttpServletResponse response)
*/
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
// TODO Auto-generated method stub
doGet(request, response);
}
}
13 css / Style.css
@charset "UTF-8";
header{
background-color: #CED46A;
border-style: solid 1px;
border-color: #CED46A;
width: 100%;
height: 80px;
line-height: 80px;
text-align: center;
color: #07553B;
}
nav{
width: 100%;
text-align: center;
height: 50px;
line-height: 50px;
background-color: #07553B;
border-style: solid 1px;
border-color: #ffefd5;
color: #CED46A;
}
section{
border-style: solid 1px;
border-color: white;
width: 100%;
height: 400px;
vertical-align: center;
text-align: center;
}
footer{
width: 100%;
text-align: center;
background-color: #07553B;
color: white;
border-style: solid 1px;
border-color: #07553B;
}
h3{
text-align: center;
}
span{color: #07553B;}
hr{border: 2px solid #CED46A;}
form{
width: 100%;
text-align: center;
}
table{
width: 100%;
text-align: center;
margin: 5px;
padding: 5px;
border-collapse: collapse;
}
th,td{
margin: 5px;
padding: 10px;
text-align: center;
}
th{
background-color: #2BAE66;
color: #FCF6F5;
}
.container{
width: 100%;
height: 400px;
}
a:link {color: #CED46A; text-decoration: none;}
a:visited {color: #CED46A; text-decoration: none;}
a:hover {color: white; text-decoration: underline;}
'STUDY > JSP' 카테고리의 다른 글
학생 성적 관리 페이지 예제 - 2021.10.05 (0) | 2023.12.09 |
---|---|
회원가입 예제 - 2021.09.29 (0) | 2023.12.09 |