* 간단한 회원가입 예제
01 데이터베이스
1) PERSON 테이블
2) ID, NAME, PW, PHONE 컬럼
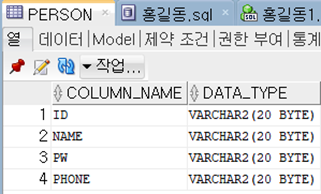
02 메인 페이지 / main.html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Main Page</title>
<link href="css/Style.css" rel="stylesheet" type="text/css"/>
</head>
<body>
<h2><span>메인</span> 홈페이지</h2>
<hr width="400px" align="left"><p>
<img src="image/bg0.jpg" width="400px" height="150px">
<table>
<tr>
<td><a href="input.html">회원가입</a>  </td>
<td><a href="list.jsp">회원목록</a>  </td>
<td><a href="login.jsp">로그인</a>  </td>
<td><a href="main.html">메인화면</a>  </td>
</tr>
</table>
</body>
</html>
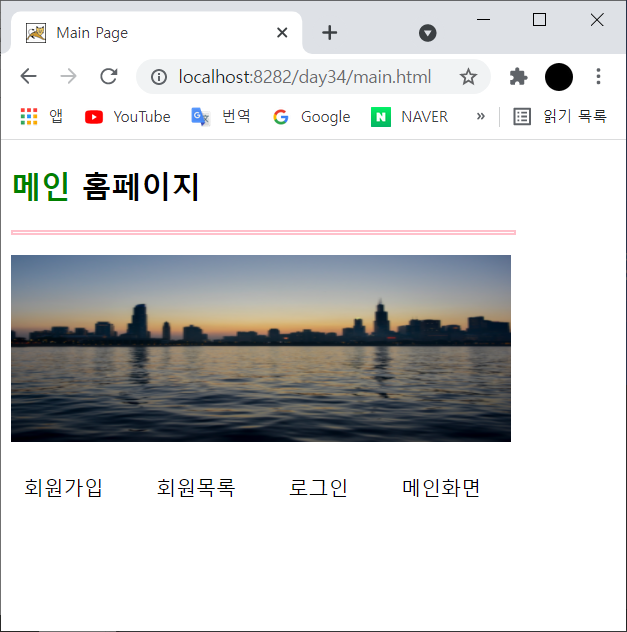
03 회원가입 / input.html
1) input.do --> servlet, 맨 밑에 모아 작성
2) 회원가입 버튼을 누르고 성공적으로 데이터베이스에 입력이 되면 로그인 창으로 이동
3) 취소를 누르면 메인 페이지로 이동
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>SignUp Page</title>
<link href="css/Style.css" rel="stylesheet" type="text/css"/>
</head>
<body>
<h2><span>회원 가입</span> 페이지</h2>
<hr width="400px" align="left"><p>
<form action="input.do" method="post">
<fieldset style="border:1; width:380px">
<table>
<tr><td>아이디</td><td><input type="text" name="id" required placeholder="아이디"></td></tr>
<tr><td>패스워드</td><td><input type="password" name="pw" required placeholder="패스워드"></td></tr>
<tr><td>이름</td><td><input type="text" name="name" required placeholder="이름"></td></tr>
<tr><td>전화번호</td><td><input type="text" name="phone" placeholder="010-0000-0000"></td></tr>
</table>
<p>
 <input type="submit" value="회원가입">
<input type="button" value="취소" onclick="location.href='main.html'">
</fieldset>
</form>
</body>
</html>

04 로그인 / login.jsp
1) login.do --> servlet
2) 취소를 누르면 메인 페이지로 이동
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Login Page</title>
<link href="css/Style.css" rel="stylesheet" type="text/css" />
</head>
<body>
<h2><span>로그인</span> 페이지</h2>
<hr width="400px" align="left"><p>
<form action="login.do" method="post">
<fieldset style="border:1; width:380px">
<table>
<tr><td>아이디</td><td><input type="text" name="s_id" required placeholder="아이디"></td></tr>
<tr><td>패스워드</td><td><input type="password" name="s_pw" required placeholder="패스워드"></td></tr>
</table>
<p>
 <input type="submit" value="로그인">
<input type="button" value="취소" onclick="location.href='main.html'">
</fieldset>
</form>
</body>
</html>
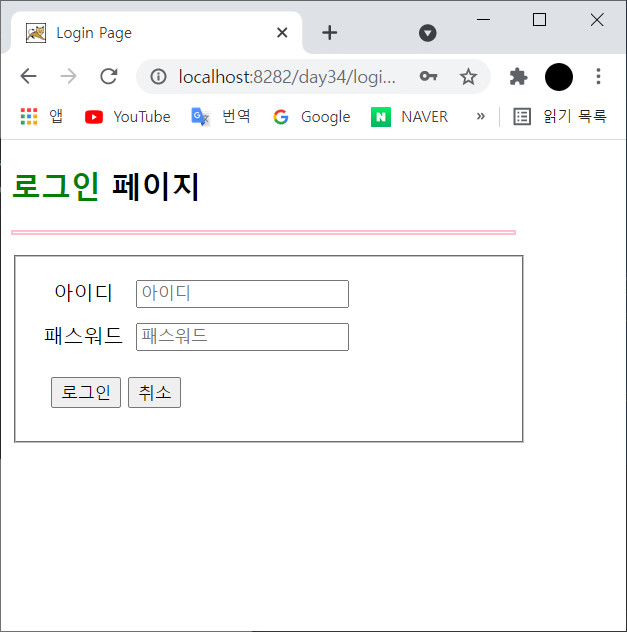
05 회원목록 / list.jsp
1) 데이터베이스에 있는 데이터들 모두 출력
2) 비고에 있는 수정 버튼을 누르면 update.jsp로 이동 (id와 name 값을 가지고 이동)
3) 메인화면으로 이동
<%@ include file="dbconnect.jsp" %>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
<link href="css/Style.css" rel="stylesheet" type="text/css"/>
</head>
<body>
<h2><span>회원 목록</span> 페이지</h2>
<hr width="400px" align="left"><p>
<%!
String id, name, pw, phone;
%>
<%
String sql = "select * from person";
psmt=con.prepareStatement(sql);
res=psmt.executeQuery();
%>
<table border="1" width="400px">
<thead>
<tr><th>ID</th><th>PW</th><th>NAME</th><th>PHONE</th><th>비고</th></tr>
</thead>
<%
while(res.next()){
String id = res.getString("id");
String pw = res.getString("pw");
String name = res.getString("name");
String phone = res.getString("phone");
%>
<tbody>
<tr><td><%=id %></td><td><%=pw %></td><td><%=name %></td><td><%=phone %></td><td><a href="update.jsp?u_id=<%=id %>&u_name=<%=name %>">수정</a></td></tr>
</tbody>
<%
}
%>
</table>
<p>
 <a href="main.html">메인화면</a>
</body>
</html>
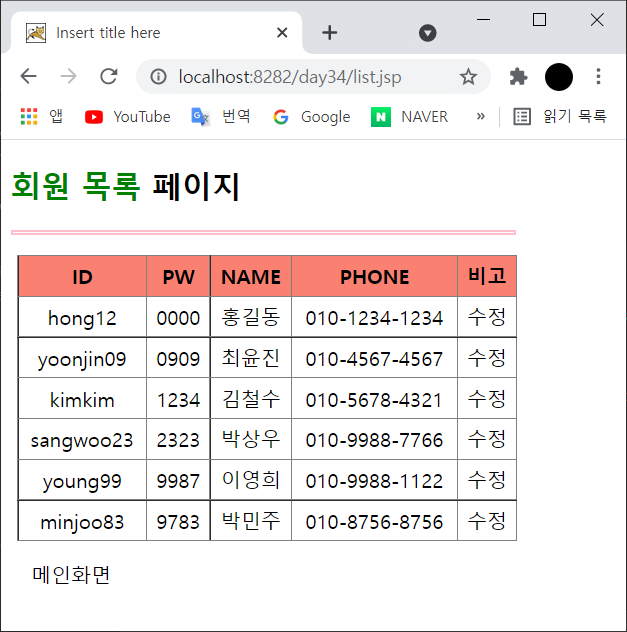
06 회원수정 / update.jsp
1) 아이디와 이름은 변경 불가, 패스워드와 전화번호만 변경 가능
2) 아이디를 HttpSession으로 저장
3) 수정 버튼을 누르면 update.do --> servlet
4) 삭제 버튼을 누르면 id 값을 가지고 delete.do로 이동 --> servlet
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Modify Page</title>
</head>
<body>
<%
String u_id = request.getParameter("u_id");
String u_name = request.getParameter("u_name");
%>
<h2><span>회원 정보 수정</span> 페이지</h2>
<hr width="400px" align="left"><p>
<form action="update.do" method="post">
<fieldset style="border:1; width:380px">
<table>
<tr><td>아이디</td><td><%=u_id %></td></tr>
<tr><td>패스워드</td><td><input type="password" name="u_pw" required placeholder="패스워드"></td></tr>
<tr><td>이름</td><td><%=u_name %></td></tr>
<tr><td>전화번호</td><td><input type="text" name="u_phone" placeholder="010-0000-0000"></td></tr>
</table>
<%
HttpSession hs = request.getSession();
hs.setAttribute("id", u_id);
%>
<p>
 
<input type="submit" value="수정">
<input type="button" value="삭제" onclick="location.href='delete.do?d_id=<%=u_id%>'">
</fieldset>
</form>
</body>
</html>

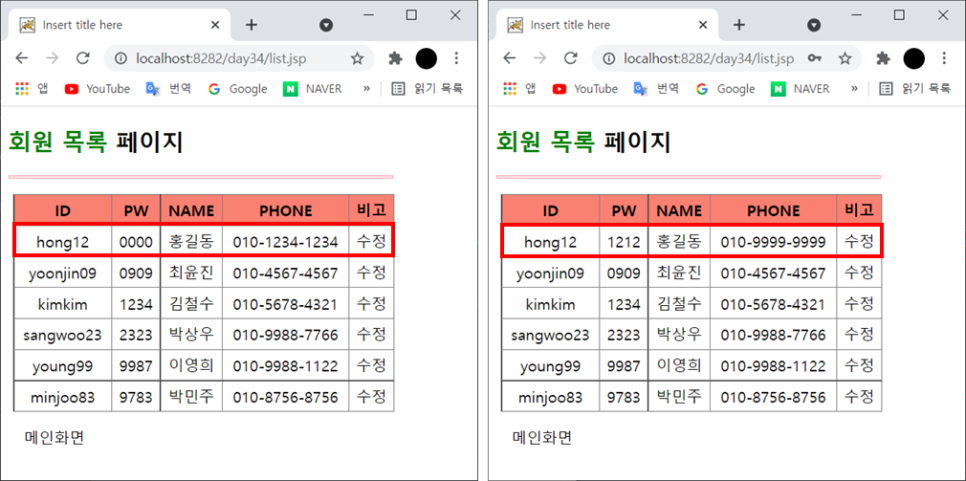
07 회원삭제

08 Servlet / TotalServlet.java
1) input.do - 회원가입
- 데이터베이스에 아이디, 패스워드, 이름, 전화번호 삽입
2) login.do - 로그인
- 데이터베이스에 아이디와 패스워드가 일치하면 로그인 성공, 메인 홈페이지로
- 데이터베이스에 아이디와 패스워드가 일치하지 않으면 로그인 실패, 회원가입 폼으로
3) update.do - 회원정보수정
- Session에서 id를 넘겨 받아 u_id에 저장
- 데이터베이스에 u_id에 일치하는 데이터의 패스워드와 전화번호 수정
4) delete.do - 회원정보삭제
- 데이터베이스에서 id를 찾아 해당 데이터 삭제 후 회원목록으로
package com.person;
import java.io.IOException;
import java.io.PrintWriter;
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import javax.servlet.http.HttpSession;
@WebServlet("*.do")
public class TotalServlet extends HttpServlet {
private static final long serialVersionUID = 1L;
public TotalServlet() {
super();
// TODO Auto-generated constructor stub
}
protected void doGet(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
request.setCharacterEncoding("utf-8");
response.setContentType("text/html;charset=utf-8");
Connection con=null;
PreparedStatement psmt;
ResultSet res;
String uri = request.getRequestURI();
String conpath = request.getContextPath();
String comm = uri.substring(conpath.length());
String driver = "oracle.jdbc.driver.OracleDriver";
String url = "jdbc:oracle:thin:@localhost:1521:xe";
try {
Class.forName(driver);
con = DriverManager.getConnection(url, "아이디", "비밀번호");
} catch (ClassNotFoundException e) {
e.printStackTrace();
} catch (SQLException e) {
e.printStackTrace();
}
if(comm.equals("/input.do")) {
String id = request.getParameter("id");
String name = request.getParameter("name");
String pw = request.getParameter("pw");
String phone = request.getParameter("phone");
String sql = "insert into person values(?,?,?,?)";
try {
psmt=con.prepareStatement(sql);
psmt.setString(1, id);
psmt.setString(2, name);
psmt.setString(3, pw);
psmt.setString(4, phone);
int k = psmt.executeUpdate();
if(k==1)
response.sendRedirect("login.jsp");
} catch (SQLException e) {
e.printStackTrace();
}
}
else if(comm.equals("/login.do")){
String s_id = request.getParameter("s_id");
String s_pw = request.getParameter("s_pw");
String sql = "select * from person where id=? and pw=?";
try {
psmt=con.prepareStatement(sql);
psmt.setString(1, s_id);
psmt.setString(2, s_pw);
res=psmt.executeQuery();
if(res.next()) { // 로그인 성공
response.sendRedirect("main.html");
}
else { // 로그인 실패
response.sendRedirect("input.html");
}
} catch (SQLException e) {
// TODO Auto-generated catch block
e.printStackTrace();
}
}
else if(comm.equals("/update.do")) {
HttpSession hs = request.getSession();
String u_id = (String)hs.getAttribute("id");
String u_pw = request.getParameter("u_pw");
String u_phone = request.getParameter("u_phone");
String sql = "update person set pw=?, phone=? where id=?";
try {
psmt=con.prepareStatement(sql);
psmt.setString(1, u_pw);
psmt.setString(2, u_phone);
psmt.setString(3, u_id);
int k = psmt.executeUpdate();
if(k==1)
response.sendRedirect("list.jsp");
} catch (SQLException e) {
e.printStackTrace();
}
}
else if(comm.equals("/delete.do")) {
request.setCharacterEncoding("utf-8");
String d_id = request.getParameter("d_id");
String sql = "delete from person where id=?";
try {
psmt=con.prepareStatement(sql);
psmt.setString(1, d_id);
int k = psmt.executeUpdate();
if(k==1)
response.sendRedirect("list.jsp");
} catch (SQLException e) {
e.printStackTrace();
}
}
}
protected void doPost(HttpServletRequest request, HttpServletResponse response) throws ServletException, IOException {
doGet(request, response);
}
}
09 DBConnect / dbconnect.jsp
1) 반복되는 데이터베이스 연결문 작성하여 <%@ include file="dbconnect.jsp" %>로 활용가능
<%@page import="java.sql.*"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>Insert title here</title>
</head>
<body>
<%
Connection con;
PreparedStatement psmt;
ResultSet res;
String driver = "oracle.jdbc.driver.OracleDriver";
String url = "jdbc:oracle:thin:@localhost:1521:xe";
Class.forName(driver);
con = DriverManager.getConnection(url, "아이디", "비밀번호");
%>
</body>
</html>
10 css 파일
1) css파일은 간단하게만 작성
@charset "UTF-8";
span{color: green}
hr{border: 2px solid pink}
table{
margin: 5px;
padding: 5px;
border-collapse: collapse;
}
th,td{
margin: 5px;
padding: 5px;
text-align: center;
}
th{
background-color: #fa8072;
}
a:link {color: black; text-decoration: none;}
a:visited {color: black; text-decoration: none;}
a:hover {color: blue; text-decoration: underline;}
'STUDY > JSP' 카테고리의 다른 글
도서 관리 페이지 예제 - 2021.10.06 (1) | 2023.12.09 |
---|---|
학생 성적 관리 페이지 예제 - 2021.10.05 (0) | 2023.12.09 |