01 예외처리
1. 인터럽트(Interrupt): 긴급 호출, 컴퓨터가 사용자에게 SOS 신호를 전송(에러가 났음을 알려줌)
(1) 하드웨어 인터럽트: 정전, 기계착오 등
(2) 소프트웨어 인터럽트: 0으로 나눈 연산, overflow, 알 수 없는 명령 등
ex) 문자열 자르기 예제
package com.test;
public class ExceptionTest {
public static void main(String[] args) {
int a,b;
String str = "023000";
a = Integer.parseInt(str.substring(0, 3));
b = Integer.parseInt(str.substring(3, 6));
System.out.println(a);
System.out.println(b);
}
}
① 7행: a,b 는 모두 int형 이므로 str을 자른 후 Integer로 형변환
[결과화면]

ex) 문자열 자르기 예제2
package com.test;
public class ExceptionTest {
public static void main(String[] args) {
int a,b;
String str = "02300a";
a = Integer.parseInt(str.substring(0, 3));
b = Integer.parseInt(str.substring(3, 6));
System.out.println(a);
System.out.println(b);
}
}
[결과화면]

① a, b는 int형인데 str에 'a'라는 문자가 들어간다면? > 오류
package com.test;
public class ExceptionTest {
public static void main(String[] args) {
int a,b;
String str = "02300a";
try {
a = Integer.parseInt(str.substring(0, 3));
b = Integer.parseInt(str.substring(3, 6));
System.out.println(a);
System.out.println(b);
} catch(NumberFormatException e) {
System.out.println("!!숫자만 입력가능합니다!!");
}
}
}
[결과화면]

* 예외처리를 이용해 한번 더 기회를 줄 수 있음
ex) 몫과 나머지 구하기 (0으로 나눴을 때)
package com.test;
public class ExceptionTest {
public static void main(String[] args) {
int a,b;
String str = "023000";
a = Integer.parseInt(str.substring(0, 3));
b = Integer.parseInt(str.substring(3, 6));
int quotient = a / b; // 몫
int remainder = a % b ; // 나머지
System.out.println("몫 = " + quotient);
System.out.println("나머지 = " + remainder);
}
}
[결과화면]

① a = 23, b = 0 일 때, a와 b를 나누면 23 나누기 0 -> 0으로 나눌 수 없다
② ArithmeticException: 에러를 잡는 클래스
2. try-catch
try{
실행문
} catch(에러를잡는클래스 e){ // e: 사용자 변수
에러 발생 시 실행문
}
package com.test;
public class ExceptionTest {
public static void main(String[] args) {
int a,b;
String str = "023000";
try {
a = Integer.parseInt(str.substring(0, 3));
b = Integer.parseInt(str.substring(3, 6));
int quotient = a / b; // 몫
int remainder = a % b ; // 나머지
System.out.println("몫 = " + quotient);
System.out.println("나머지 = " + remainder);
}catch (ArithmeticException e) {
System.out.println("!!0으로 나눌 수 없습니다!!");
}catch (NumberFormatException e) {
System.out.println("!!숫자만 입력가능합니다!!");
}
}
}
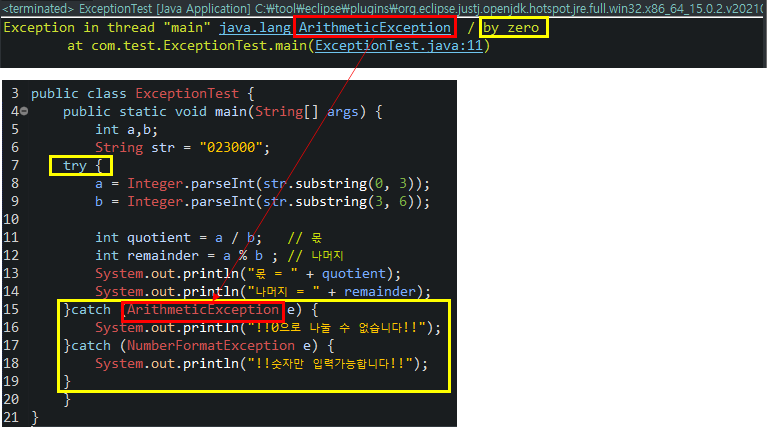
[결과화면]

3. Exception: 예외 처리 클래스 중에서 가장 순위가 높은 클래스 > 가장 맨 뒤에 쓸 것
package com.test;
public class ExceptionTest {
public static void main(String[] args) {
int a,b;
String str = "023000";
try {
a = Integer.parseInt(str.substring(0, 3));
b = Integer.parseInt(str.substring(3, 6));
int quotient = a / b; // 몫
int remainder = a % b ; // 나머지
System.out.println("몫 = " + quotient);
System.out.println("나머지 = " + remainder);
}catch (ArithmeticException e) {
System.out.println("!!0으로 나눌 수 없습니다!!");
}catch (NumberFormatException e) {
System.out.println("!!숫자만 입력가능합니다!!");
}catch (Exception e) {
System.out.println(e.getMessage());
}
}
}
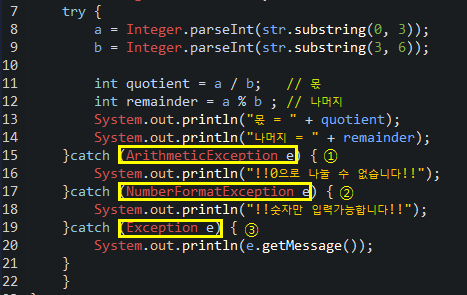
* ①, ② 에서 못 잡은 에러를 ③에서 잡아줄 수 있음
4. 사용자 예외처리
* 예외 처리 종류 (1) Exception (2) throws(던지다) -> 에러가 발생한 메소드 쪽으로 던진다는 의미
ex) 5개의 홀수 구하기 (짝수일 때 예외처리 하기)
package com.test;
import java.util.Scanner;
class MyException extends Exception{
public MyException() {
super();
}
}
public class ExceptionTest {
public static void main(String[] args) throws Exception {
Scanner sc = new Scanner(System.in);
int a;
for(int i=0; i<5; i++) {
try {
System.out.print((i+1) + "번째 두자리 수를 입력하세요: ");
String temp = sc.nextLine();
a = Integer.parseInt(temp.substring(0, 2));
if(a % 2 == 0) { // 짝수이면
i--;
throw new MyException();
}
else
System.out.println("입력된 수는 " + a + "입니다.");
}catch (NumberFormatException e) {
System.out.println("!!문자가 입력되었습니다!!");
i--;
}catch (MyException e) {
System.out.println("!!홀수만 입력하세요!!");
}catch (Exception e) {
System.out.println(e.getMessage());
}
}
}
}
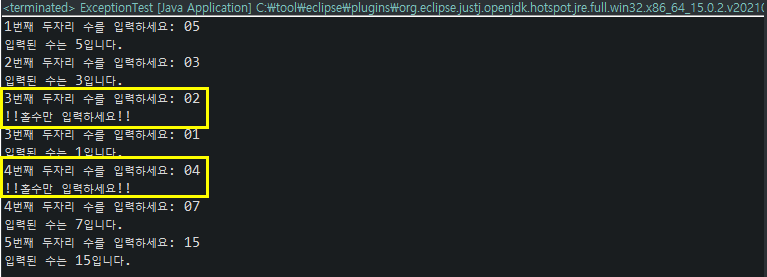
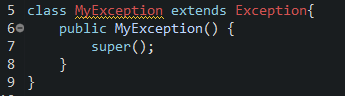
① 사용자 예외처리 클래스 생성
② default 생성자 생성
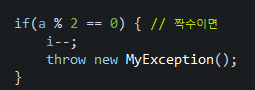
③ 예외처리 만들 구간에 thorw new MyException 호출
④ 5개의 홀수를 구하기 위해 짝수이면 횟수 차감

④ throws Exception 선언
'STUDY > Java' 카테고리의 다른 글
[JAVA] Generic 제네릭 (0) | 2021.11.05 |
---|---|
[JAVA] Thread, 스레드 (0) | 2021.11.05 |
[JAVA] 정렬, 익명클래스 (0) | 2021.11.05 |
[JAVA] 데이터 타입 - 기본타입, 참조타입 (0) | 2021.11.05 |
[JAVA] 추상 클래스, 인터페이스 (0) | 2021.11.05 |